# Turtle Library
We start learning python in a fun way by using python turtle graphic library.
#
Codemao Python Online Editor (opens new window)
# Basic
#
Firstly we import turtle library
from turtle import *
# turtle is the library
# * is the global variable
move forward
fd() # let turtle forward any step
eg: fd(100) # forward 100 steps
move backward
bk() # let turtle backward any step
eg: bk(100) # backward 100 steps
turn right
rt() # rotate right any degree
eg: rt(90) # rotate right 90 degree
turn left
lt() # rotate left any degree
eg: lt(90) # rotate left 90 degree
draw a circle
cricle()
# value is radius and it can be positive or negative integer(20,30, -33...) or decimal(9.3, -32.33...)
circle(100)
# if the value is positive, it's a anticlockwise circle
circle(40.56)
# if the value is negative, it's a clockwise circle
set pen color
() # set pen color
eg1: color('red') # set pen color by color name
eg2: color(119, 171, 170) # set pen color by RGB value
eg3: color('#77abaa') #s et pen color by color code
set background color
bgcolor() # set background color
eg: bgcolor('green') # set background color green
set turtle moving speed
speed() # set turtle moving speed
speed(10)
# The number can be from 1 to 10 and has to be a whole number.
# You cannot have for example 4.7 as the number.
# Setting it above 10 will cause the speed to not work.
set pen size
pensize()
pensize(5)
go to the specic position
goto(x, y)
# x and y are coordinates in the graphic
fill color
fillcolor('blue') # set filling color
begin_fill() # set current position as the beginning position to fill in color
... code here ...
end_fill() # set current position as the end position to finish filling
fillcolor('red')
begin_fill()
circle(90)
end_fill()
pen up & pen down
penup() # pen up - not drawing
pendown() # pen down - drawing
clear all drawing
clear()
draw word
write('Hello')
#
Exercise 1: Draw a running windmill with 8 parts.
#
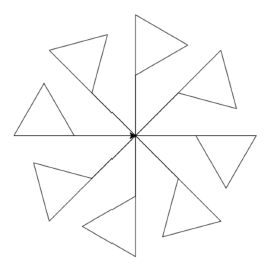
# solution:
speed(100)
for i in range(8):
fd(100)
for i in range(3):
fd(100)
rt(120)
bk(100)
rt(45)
# Repeat
#
for loop
for i in range(10):
print(i)
#repeat 10 times
# 4 spaces indent
# i is the changing variable which can be any name
# i changes from 0 to 9 which is 0->1->2->3->4->5->6->7->8->9
for i in range(2,4):
print(i)
#i changes from 2 to 3 which is 2->3
for i in range(2,10,3):
print(i)
#i changes from 2 to 9 by 3 which is 2->5->8
#Don't forget semicolon and indent(4 spaces)
# Function
#
function - draw a square
def square():
for i in range(4):
fd(100)
rt(90)
function - draw a triangle
def tri():
for i in range(3):
fd(100)
rt(120)
function - draw a polygon
def poly(n):
for i in range(n):
fd(30)
lt(360/n)
main() function
if __name__ == "__main__": #python syntax for main() function entrance
square()
traingle()
poly()
#
DIY your own function
#
Exercise 2: Draw 27 polygons from triangle to the polygon with 30 edges.
#
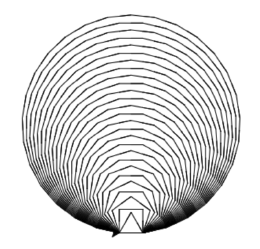
# solution:
def poly(n):
for i in range(n):
fd(30)
lt(360/n)
def pic():
for i in range(3,30):
poly(i)
pic()